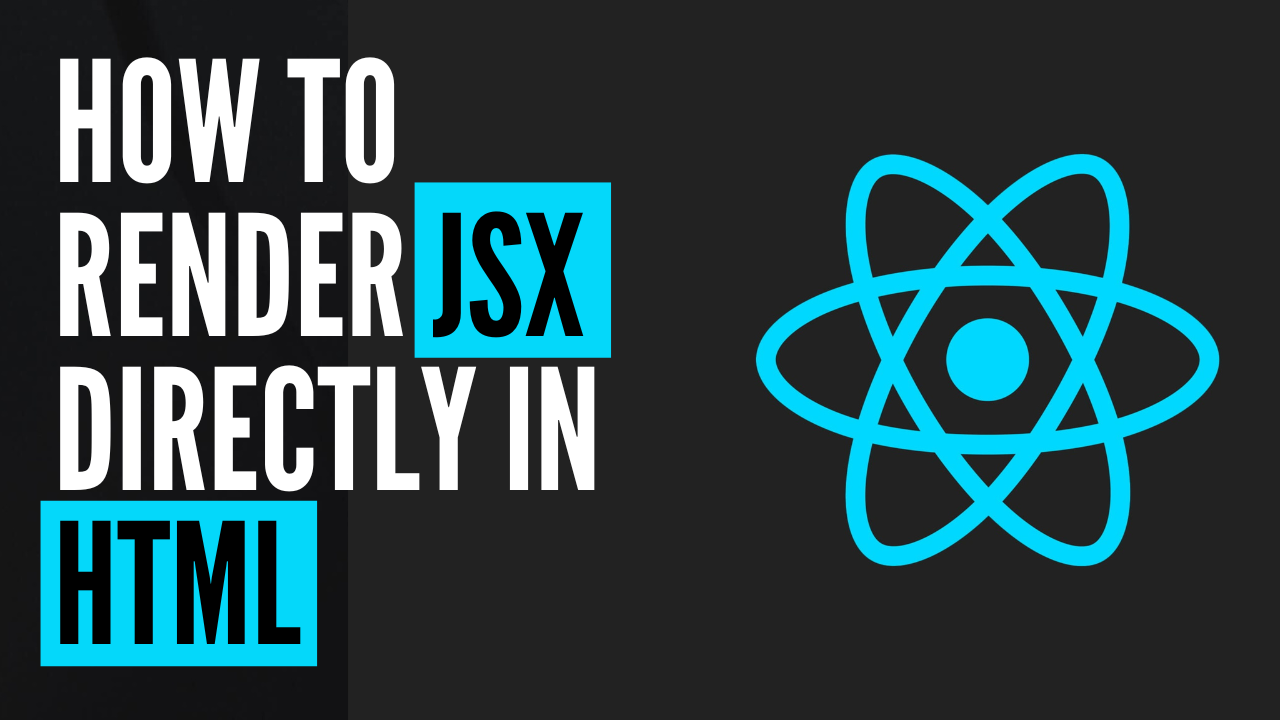
How to render JSX in HTML
In this tutorial, you will learn how to render JSX in HTML.
Video tutorial:
Include JS files
First, you need to include the required JS files. The required JS files are:
- React
- React DOM
- Babel
You can download all these files from cdnjs.com. Make sure to include the UMD version and not CJS.
- CJS: Common JS
- UMD: Universal Module Definition
You might like:
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/18.2.0/umd/react.development.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/18.2.0/umd/react-dom.development.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-standalone/7.23.10/babel.js"></script>
Render JSX
The following code will render the JSX code directly in HTML.
<div id="app"></div>
<script type="text/babel">
function MyApp() {
return (
<h1>Hello JSX</h1>
)
}
ReactDOM.createRoot(
document.getElementById("app")
).render(<MyApp />)
</script>
Make sure to set the type of script tag as “text/babel”.
Render JSX from an external JS file
To render JSX from an external JS file, you first need to include the JS file with a script tag having type as “text/babel”.
<script type="text/babel" src="js/MyApp.js"></script>
Then your “js/MyApp.js” file will look like this.
// src/MyApp.js
function MyApp() {
return (
<h1>Hello JSX</h1>
)
}
ReactDOM.createRoot(
document.getElementById("app")
).render(<MyApp />)
Check out our more tutorials on React JS. If you face any problem in following this, kindly do let me know.