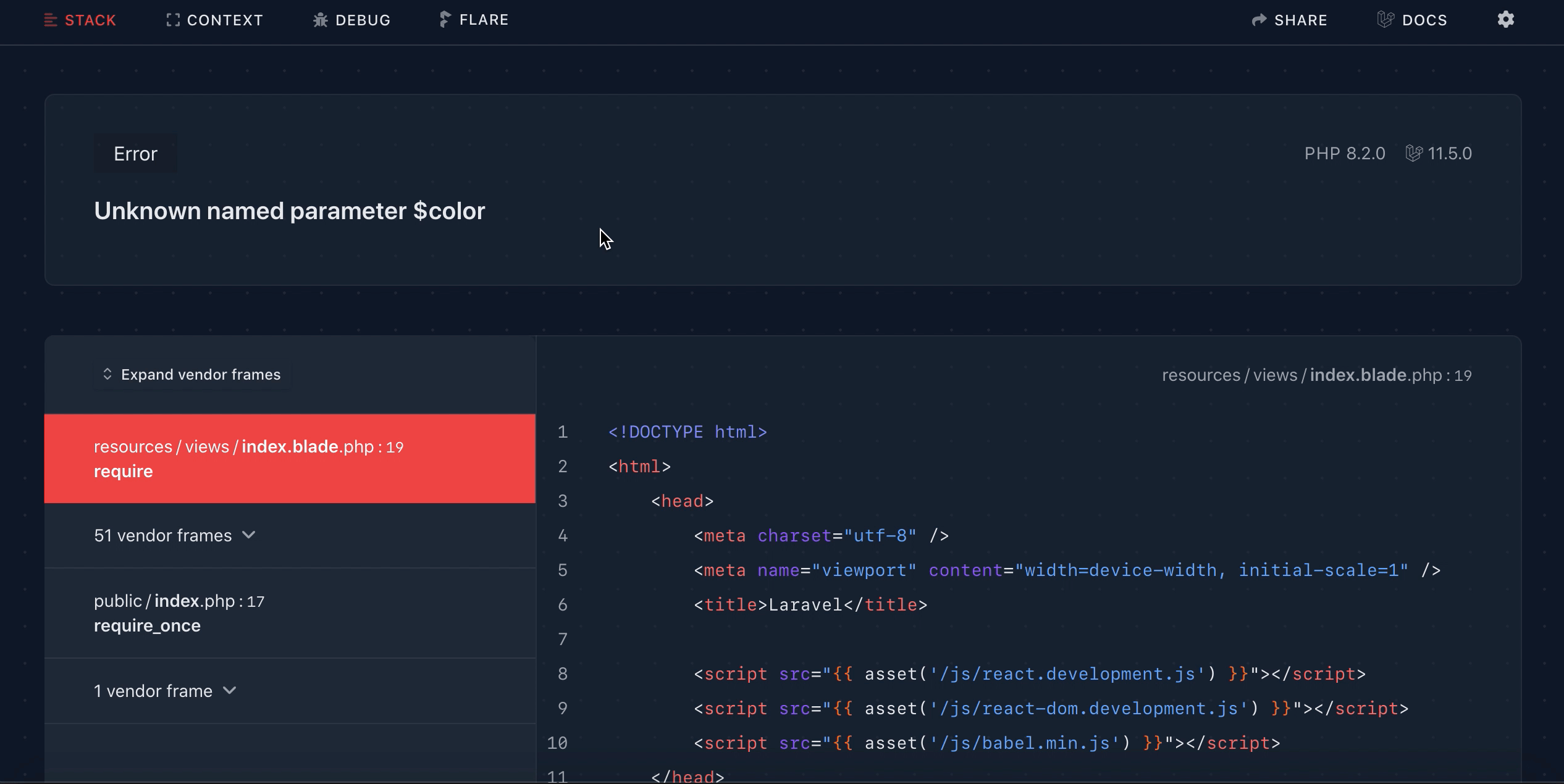
Use React JS styles in Laravel blade template
If you are trying to give CSS styles in React JS inside Laravel blade template, but having the following error:
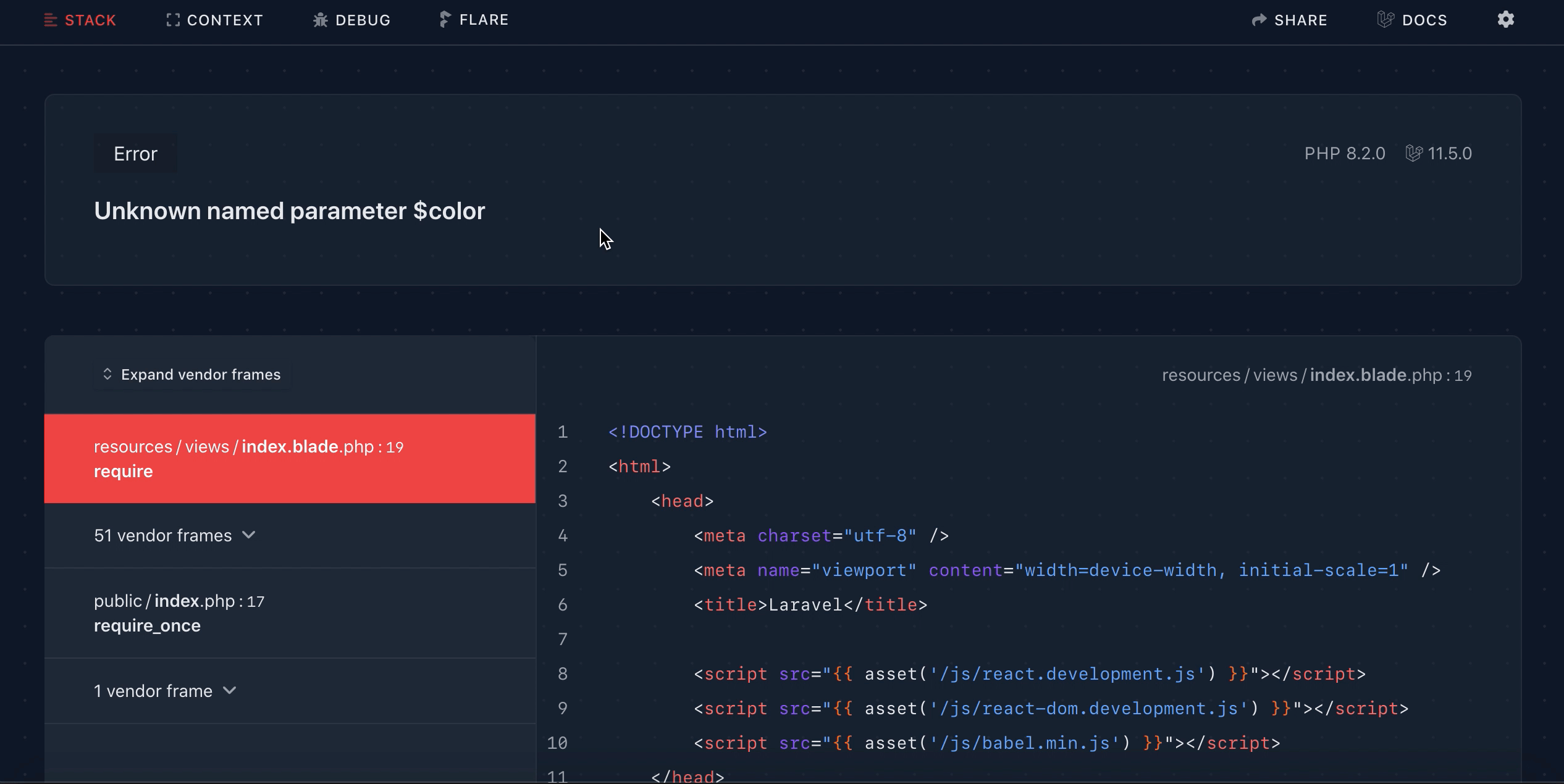
This happens when you try to give CSS styles in React JS in the following way:
function MyApp() {
return (
<h1 style={{
color: "red"
}}>Hello world</h1>
)
}
ReactDOM.createRoot(
document.getElementById("react-app")
).render(<MyApp />)
The error you are having is because the double curly braces {{ which are used in React JS for styling the tag, are also used by Laravel blade template to render the value of a PHP variable. Laravel will think of it as a PHP variable and will throw an error.
To fix this error, you need to create a separate object in your React JS component. That object will have all the styles for each tag. Modify your React JS component to the following:
function MyApp() {
const styles = {
heading: {
color: "red"
}
}
return (
<h1 style={ styles.heading }>Hello world</h1>
)
}
You can see that instead of setting the CSS directly in the style attribute, I have created a separate variable and used its value as a variable. This way your error gets fixed. Also, it will help you in setting CSS styles to multiple tags without duplicating them.