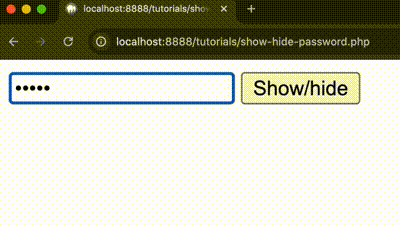
Show or hide input field password – HTML and Javascript
In this blog post, we will discuss, how you can show or hide an input field for password using plain HTML and Javascript. This is useful because sometimes users wants to see what password they are setting. This feature is very helpful in:
- Signup, because user will be entering his password for the first time.
- Reset, because user wants to know what new password he is setting-up.
No library has been used in this tutorial.
First, we will create an input field for password.
<!-- input field for password -->
<input type="password" id="password" />
Then, we will create a button that will show or hide the password.
<!-- button to toggle show/hide -->
<button type="button" onclick="togglePassword()">Show/hide</button>
Finally, we will create a Javascript function that will change the type of password input field.
// function to toggle password
function togglePassword() {
// get password input field
const password = document.getElementById("password")
// change input type to password if currently is text, and change to text if currently is password
password.type = (password.type == "password") ? "text" : "password"
}
This function will first get the DOM of input field for password. Then it will execute a ternary operator to check if the current type is “password”, meaning the password is hidden, then it will set it’s type to “text” and the password will be visible to the user.
And if currently the type is “text”, it means that user is currently viewing the password, then it will set it’s type to “password” and hence hide the password.
Complete source code:
<!-- input field for password -->
<input type="password" id="password" />
<!-- button to toggle show/hide -->
<button type="button" onclick="togglePassword()">Show/hide</button>
<script>
// function to toggle password
function togglePassword() {
// get password input field
const password = document.getElementById("password")
// change input type to password if currently is text, and change to text if currently is password
password.type = (password.type == "password") ? "text" : "password"
}
</script>
Above code will create an input field and a button. By default, what you enter in the input field will not be visible to you. But once you press the button, the password will be visible. And on again pressing the button, it will be hidden. That’s how you can show or hide an input field for password using simple HTML and Javascript.