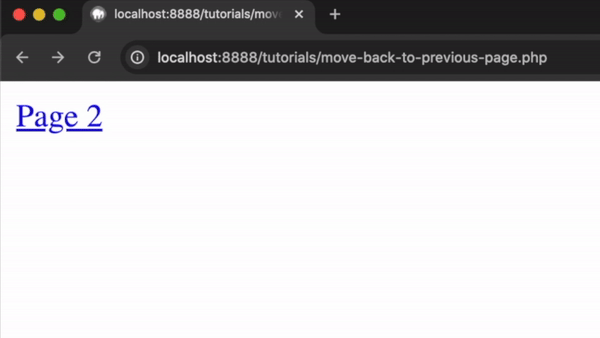
Redirect to previous page – Javascript
Let’s say you have a webpage where you want to show an anchor link to the user that will redirect the user to his previous page. So we will first create an anchor link to the first page.
<!-- create an anchor tag to page 2 and attach an onclick event listener to it -->
<a href="page2.php" onclick="gotoPage()">Page 2</a>
When it is clicked, we will call a Javascript function instead of directly redirecting to new page.
function gotoPage() {
// prevent the page from redirecting
event.preventDefault()
// get current page location and save it in local storage
localStorage.setItem("referrer", window.location.href)
// redirect to the href attribute
window.location.href = event.target.getAttribute("href") || ""
}
This function will first prevent the page from redirecting to anchor href attribute. Then it will get the current page URL, along with all its query parameters, and save it in local storage. Finally, it will get the anchor tag’s href attribute and redirect the user to it.
In order to redirect the user back to previous page, we need to create a file named “page2.php” and create another anchor link in it. By default, the anchor link will be hidden. It will only be visible if local storage has some referrer value.
<!-- create back button, hidden by default -->
<a id="referrer" style="display: none;">Go back</a>
After that, we run a Javascript code that will get the local storage value and set in to that anchor tag.
// check if value exists in referrer local storage
const referrerValue = localStorage.getItem("referrer")
if (referrerValue) {
// if yes, then set it to the href attribute of back button
const referrerNode = document.getElementById("referrer")
referrerNode.setAttribute("href", referrerValue)
// and display the button
referrerNode.style.display = ""
}
It will first get the referrer value from local storage. If there is any value, then it will get the back button node and referrer value. It will set the href attribute of back button to the referrer value. And finally, it will display the back button. If there is no referrer, then the back button will stay hidden.