I created an online free FTP manager in PHP that allows developers to work on their projects from anywhere. I created this tool in PHP and MySQL using Laravel framework. The frontend is designed in Bootstrap and React JS.
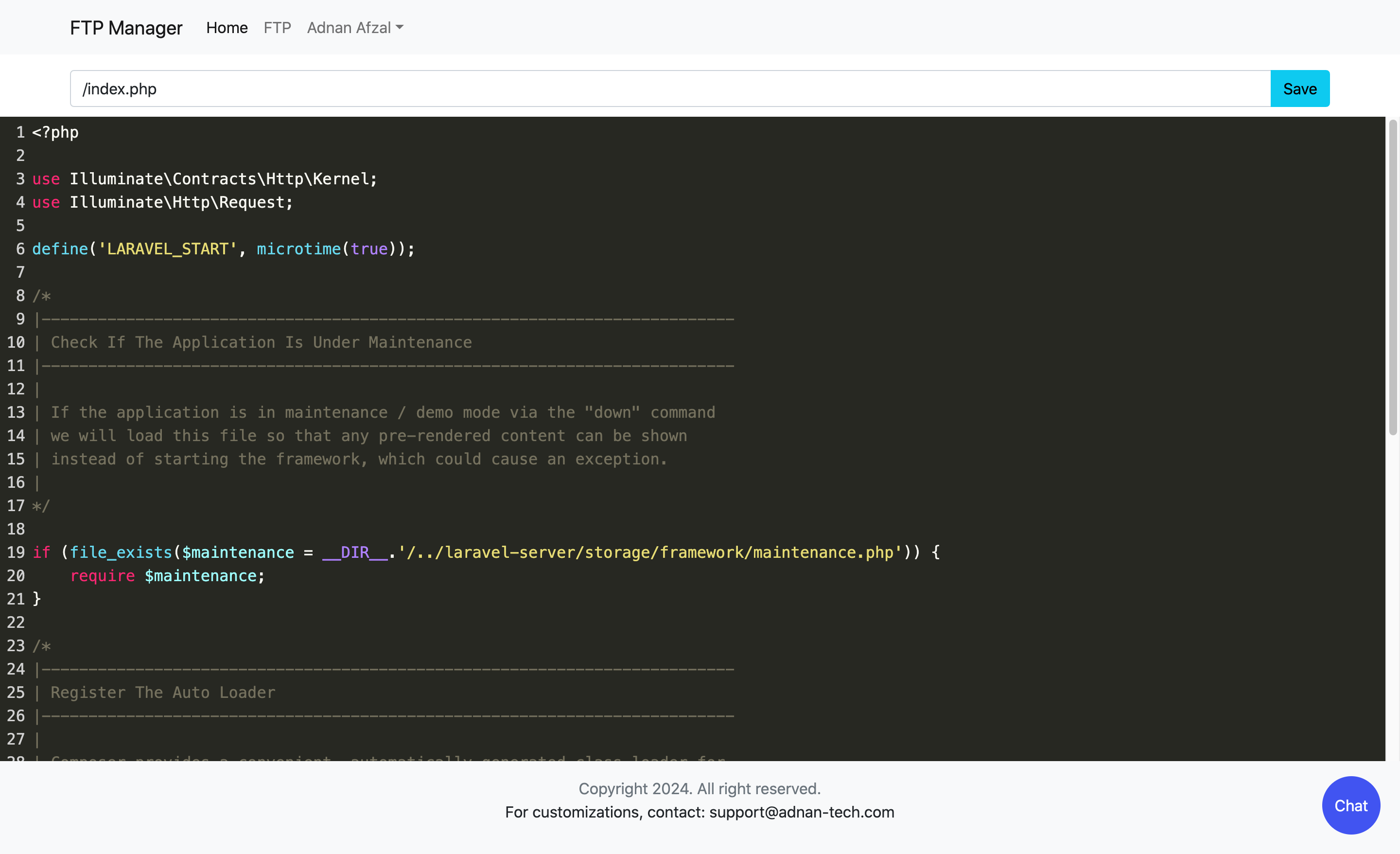
Let’s discuss each feature and I will also show you how I built this.
What you will learn:
- Connect with FTP.
- List files from FTP directory.
- Fetch file content.
- Edit FTP file.
- Create a new file.
- Create a new folder.
- Upload files.
- Download files.
- Rename file.
- Delete file.
- Delete folder.
We created a PHP class named “FTP” in a file called “FTP.php”. All our functions will go in that class.
1. Connect with FTP
The first step is to connect with FTP server. Following code will create a function that will connect with your FTP server (we are not calling it yet).
class FTP
{
private $conn_id = null;
private $server = "";
private $username = "";
private $password = "";
private function connect()
{
try
{
// Establishing connection
$this->conn_id = ftp_connect($this->server);
if (!$this->conn_id)
{
echo json_encode([
"status" => "error",
"message" => "Could not connect to " . $this->server
]);
exit();
}
// Login with username and password
$login_result = ftp_login($this->conn_id, $this->username, $this->password);
if (!$login_result)
{
echo json_encode([
"status" => "error",
"message" => "Wrong password for '" . $this->username . "'."
]);
exit();
}
// Enable passive mode for better compatibility
ftp_pasv($this->conn_id, true);
}
catch (\Exception $exp)
{
echo json_encode([
"status" => "error",
"message" => "Could not connect to " . $this->server
]);
exit();
}
}
}
Comments has been added with each line for explanation. Make sure to enter your correct FTP server address, FTP account’s username and its password. In the next step, we will call this function to connect with FTP server.
2. List files from FTP directory
The next step is to list all files from FTP directory. Create the following function in your FTP class.
public function fetch_files()
{
$this->do_connect();
$path = "/directory-name";
// Get file listing
$file_list = ftp_nlist($this->conn_id, $path);
if ($file_list === false)
{
echo json_encode([
"status" => "error",
"message" => "Error retrieving file list."
]);
exit();
}
$files = [];
foreach ($file_list as $file)
{
$obj = [
"file" => $file,
"size" => ftp_size($this->conn_id, $file)
];
if ($obj["file"] != "." && $obj["file"] != "..")
{
array_push($files, $obj);
}
}
// Close the connection
ftp_close($this->conn_id);
echo json_encode([
"status" => "success",
"message" => "Data has been fetched.",
"files" => $files
]);
exit();
}
- This function will first connect with the FTP server.
- Then it will fetch all the files from $path variable.
- If the directory does not exists or if it does not have read permission, then it will return an error.
- All files from that directory will be put in an array along with the size of the file in bytes.
- If it is a directory, then it’s size will be -1.
- Finally, it returns the array with the response.
3. Fetch file content
Reading the content of FTP file is necessary because in order to edit the file, we need to first view the file. Following function will fetch the content of file and return it in response.
public function fetch_content()
{
$this->do_connect();
$file = "/directory-name/file-name.php";
// Local path for downloading the file
$local_file = tempnam(sys_get_temp_dir(), 'ftp_download');
// Download the file from FTP server to local file
if (!ftp_get($this->conn_id, $local_file, $file, FTP_BINARY))
{
echo json_encode([
"status" => "error",
"message" => "Failed to read '" . $file . "'"
]);
exit();
}
// Read contents of the local file
$file_content = file_get_contents($local_file);
// Display or process the file content as needed
if ($file_content === false)
{
echo json_encode([
"status" => "error",
"message" => "Failed to read file content."
]);
exit();
}
// Clean up: Delete the temporary local file
unlink($local_file);
echo json_encode([
"status" => "success",
"message" => "Data has been fetched.",
"content" => $file_content
]);
exit();
}
- It will first download the file in a temporary directory to your server.
- If the file does not exists, then it will return an error.
- It will return an error if it fails to read the FTP file.
- Usually it is because if the $path variable is a directory or an image or document file.
- After the file’s content is fetched, we will remove the temporary file from our server.
4. Edit FTP file
After the file’s content is successfully fetched, the next step is to update the file. You are working in FTP, editing the code is what you will be doing most of the time.
Updating the content of file requires 2 steps:
- Saving the file in temporary folder.
- Uploading the temporary file on FTP server.
public function update_content()
{
$this->do_connect();
$file = "/directory-name/file-name.php";
$content = "console.log(\"Hello world\")";
// Local path for downloading the file
$local_file = tempnam(sys_get_temp_dir(), 'ftp_download');
// Write the new content to a local temporary file
file_put_contents($local_file, $content);
if (!ftp_put($this->conn_id, $remote_file, $local_file, FTP_ASCII))
{
echo json_encode([
"status" => "error",
"message" => "There was a problem updating '" . $remote_file . "'"
]);
exit();
}
unlink($local_file);
// Close the FTP connection
ftp_close($this->conn_id);
echo json_encode([
"status" => "success",
"message" => "File has been updated."
]);
exit();
}
5. Create a new file
Creating a new file in FTP in PHP is as simple as updating the file. We just need to set the content of file as empty string. So we will be uploading an empty file to FTP server.
public function create_file()
{
$this->do_connect();
$path = "/directory-name";
$name = "file-name.php";
// Local file content to be uploaded
$file_content = "";
// Local path for downloading the file
$local_file = tempnam(sys_get_temp_dir(), 'ftp_download');
// Write the content to a temporary local file
file_put_contents($local_file, $file_content);
// Remote file path where the file will be created
$remote_file = $path . "/" . $name; // Replace with the desired remote file path
// Upload the local file to the FTP server
if (!ftp_put($this->conn_id, $remote_file, $local_file, FTP_ASCII))
{
echo json_encode([
"status" => "error",
"message" => "There was a problem while creating '" . $name . "'"
]);
exit();
}
unlink($local_file);
// Close the FTP connection
ftp_close($this->conn_id);
echo json_encode([
"status" => "success",
"message" => "Successfully created '" . $name . "'"
]);
exit();
}
- Create a temporary empty file on local directory.
- Upload it to FTP.
- Remove the file from local directory.
6. Create a new folder
Creating a folder is must easier than creating a file on FTP server.
public function create_folder()
{
$this->do_connect();
$path = "/directory-name";
$name = "folder-name";
$folder = $path . "/" . $name;
if (!ftp_mkdir($this->conn_id, $folder))
{
echo json_encode([
"status" => "error",
"message" => "There was a problem while creating '" . $name . "'"
]);
exit();
}
echo json_encode([
"status" => "success",
"message" => "Successfully created '" . $name . "'"
]);
exit();
}
Note: It will return an error if the directory already exists.
7. Upload files
To upload the file from local computer to FTP server, you need to first save it in your local server. Then upload the file to FTP server. After it is successfully uploaded, you can remove the file from local server.
public function upload()
{
$this->do_connect();
$path = "/directory-name";
$file_name = basename($_FILES["file"]["name"]);
$target_file = "uploads/" . $file_name;
if (!move_uploaded_file($_FILES["file"]["tmp_name"], $target_file))
{
echo json_encode([
"status" => "error",
"message" => "The file " . htmlspecialchars(basename( $_FILES["file"]["name"])) . " has been uploaded."
]);
exit();
}
if (!ftp_put($this->conn_id, $file_name, $target_file, FTP_ASCII))
{
echo json_encode([
"status" => "error",
"message" => "There was a problem updating '" . $file_name . "'"
]);
exit();
}
// Close the FTP connection
ftp_close($this->conn_id);
echo json_encode([
"status" => "success",
"message" => "File(s) has been uploaded."
]);
exit();
}
If you are working in Laravel, your upload function should be like this:
public function upload()
{
$this->do_connect();
$path = "/directory-name";
$files = request()->file("files");
foreach ($files as $file)
{
$remote_file = $file->getClientOriginalName();
$file_path = "ftp/" . $file->getClientOriginalName();
$file->storeAs("/private", $file_path);
if (!ftp_put($this->conn_id, $remote_file, storage_path("app/private/" . $file_path), FTP_ASCII))
{
return response()->json([
"status" => "error",
"message" => "There was a problem updating '" . $remote_file . "'"
]);
}
Storage::delete("private/" . $file_path);
}
// Close the FTP connection
ftp_close($this->conn_id);
return response()->json([
"status" => "success",
"message" => "File(s) has been uploaded."
]);
}
8. Download files
Downloading the file to local computer requires 2 steps.
- First is to download the file on local server from FTP server.
- Then download it to local computer from local server.
Download file from FTP to local server
Following code will be used to download the file on local server.
public function download()
{
$this->do_connect();
$path = "/directory-name";
$file = "file-name.php";
// Local path for downloading the file
$local_file = tempnam(sys_get_temp_dir(), 'ftp_download');
$remote_file = $path . "/" . $file;
// Download the file from FTP server to local file
if (!ftp_get($this->conn_id, $local_file, $remote_file, FTP_BINARY))
{
echo json_encode([
"status" => "error",
"message" => "Failed to download '" . $remote_file . "'"
]);
exit();
}
// Create a unique download URL for the file
$download_url = url('download.php?file=' . urlencode($local_file) . '&name=' . urlencode($file));
// Close the FTP connection
ftp_close($this->conn_id);
echo json_encode([
"status" => "success",
"message" => "File has been downloaded.",
"download_url" => $download_url
]);
exit();
}
It will also return the “download_url” variable. That will be link to file from where you can download the file.
Download from server to local computer
Create a new file named “download.php” in your server and write the following code in it:
if (isset($_GET['file']) && isset($_GET['name']))
{
$temp_file = $_GET['file'];
$file_name = $_GET['name'];
if (file_exists($temp_file))
{
header('Content-Description: File Transfer');
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename="' . $file_name . '"');
header('Expires: 0');
header('Cache-Control: must-revalidate');
header('Pragma: public');
header('Content-Length: ' . filesize($temp_file));
// Read the file and output it to the browser
readfile($temp_file);
// Delete the temporary file
unlink($temp_file);
exit();
}
echo "File does not exist.";
exit();
}
echo "Invalid request.";
exit();
9. Rename file
Renaming the file on FTP using PHP is very easy. You just have to provide 3 things:
- Folder name
- Old file name
- New file name
public function rename()
{
$this->do_connect();
$path = "/directory-name";
$name = "new-file-name.php";
$file = "old-file-name.php";
// File to be renamed on the FTP server
$remote_file = $path . "/" . $file;
$remote_new_file = $path . "/" . $name;
// Try to rename the file or folder
if (!ftp_rename($this->conn_id, $remote_file, $remote_new_file))
{
echo json_encode([
"status" => "error",
"message" => "There was a problem renaming " . $file . " to " . $name
]);
exit();
}
echo json_encode([
"status" => "success",
"message" => "Successfully renamed " . $file . " to " . $name
]);
exit();
}
10. Delete file
Deleting a file from FTP in PHP is very simply. You just need to enter the full path of the file and call the PHP built-in ftp_delete function. Pass the FTP connection ID and file path as arguments.
public function delete_file()
{
$this->do_connect();
$path = "/directory-name/file-name.php";
// Delete the file on the FTP server
if (!ftp_delete($this->conn_id, $path))
{
echo json_encode([
"status" => "error",
"message" => "There was a problem while deleting " . $path
]);
exit();
}
echo json_encode([
"status" => "success",
"message" => "Successfully deleted " . $path
]);
exit();
}
11. Delete folder
Deleting a folder is NOT as simple as deleting a single file. There are 2 steps involved in deleting a folder from FTP in PHP.
- Recursively delete all files and folders inside that folder.
- Delete the folder itself.
So first we will create a function that will initialize the call to recursive function.
public function delete_folder()
{
// Set maximum execution time to unlimited (0 means no limit)
set_time_limit(0);
$path = "/directory-path/folder-name";
$this->do_connect();
$this->ftp_delete_dir($path);
echo json_encode([
"status" => "success",
"message" => "Folder has been deleted."
]);
exit();
}
Our recursive function ftp_delete_dir will look like this:
// Function to recursively delete a directory
private function ftp_delete_dir($path)
{
// Get the list of files in the directory
$files = ftp_nlist($this->conn_id, $path);
// Loop through each file
foreach ($files as $file)
{
$file = basename($file);
if ($file == '.' || $file == '..')
{
continue;
}
$file_path = "$path/$file";
// If it's a directory, delete recursively
if (@ftp_chdir($this->conn_id, $file_path))
{
ftp_chdir($this->conn_id, '..');
ftpDelete($this->conn_id, $file_path);
}
else
{
// If it's a file, delete it
ftp_delete($this->conn_id, $file_path);
}
}
// Finally, remove the directory itself
ftp_rmdir($this->conn_id, $path);
}
It does the following things:
- Loops through all the files inside that folder.
- Skip the “.” and “..” folders (they are just pointing towards current and parent directory respectively).
- If the file is a directory, start the recursion.
- Else delete the file.
- Finally at line #33, we are deleting the current directory itself.
So these are all the features we have in FTP manager I created in PHP and MySQL using Laravel framework. If you have any quetions regarding FTP or if you are having any problem, feel free to contact me.